Advertisement Legacy 패키지 다운로드 받기
Advertisement Legacy 설치를 합니다.
Configure 클릭
제품명, 본인의 유니티 아이디, 프로젝트 아이디 등등의 정보들이 나옵니다.
위와 같이 뜨지 않는다면?▼

Unity Hub에 들어가셔서 해당 프로젝트의 CLOUD 부분이 CONNECTED로 되어있지 않은지 확인해보세요.
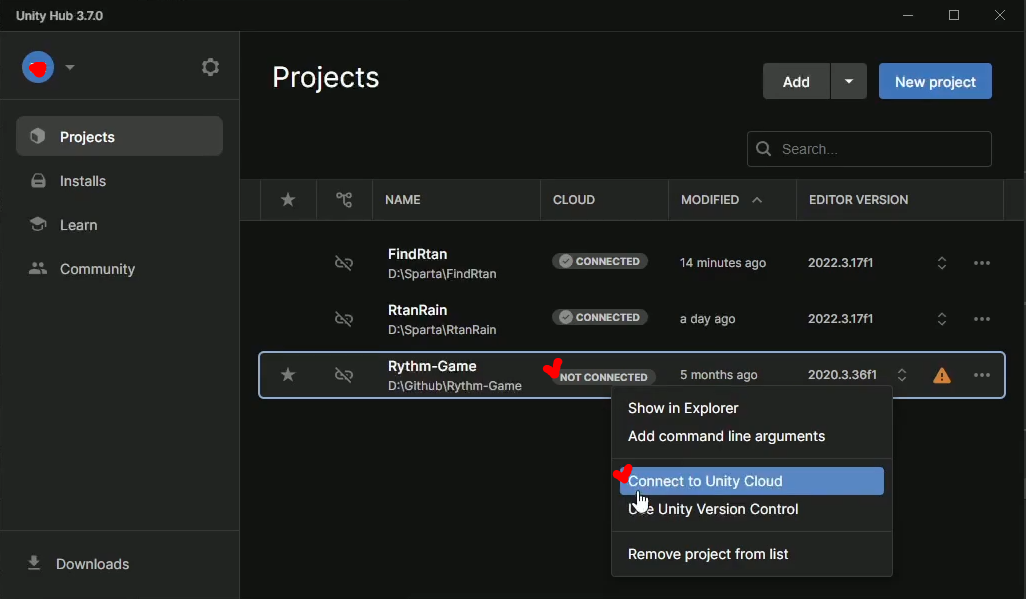
만약 NOT CONNECTED 상태라면 'Connect to Unity Cloud'를 클릭해주세요.
Unity Ads Monetization 설정하기
Dashboard 클릭
Unity 사이트가 뜨면 로그인을 합니다.
Unity Cloud로 접속하게 됩니다. Sign in 해주세요.
'Unity Ads Monetization' 을 추가합니다.
Unity Ads Monetization을 클릭하고 광고 활성화를 클릭합니다.
저는 개인 사용자라서 개인으로 체크했습니다.
'I only plan to use Unity Ads' 체크해주고 Next
아직 앱을 출시한게 아니기 때문에 '앞으로 출시할거다' 체크
애플과 안드로이드에서 출시할 수 있는 키값이 발급됨
키값을 잊어버렸다면 설정에 들어가서 다시 확인가능
Unity Ads를 어떻게 활용할 수 있는지에 대한 가이드 문서 클릭
아까 설치한 Unity Ads Monetization가 SDK입니다.
그럼 다음은 SDK 초기화를 시켜줘야하네요.
Unity Ads Monetization SDK 초기화 해주기
초기화는 유니티 문서에 나온 코드 그대로 가져다 사용해봅시다.
코드▼
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Advertisements;
public class AdInitialize : MonoBehaviour, IUnityAdsInitializationListener
{
[SerializeField] string _androidGameId;
[SerializeField] string _iOSGameId;
[SerializeField] bool _testMode = true;
private string _gameId;
void Awake()
{
InitializeAds();
}
public void InitializeAds()
{
#if UNITY_IOS
_gameId = _iOSGameId;
#elif UNITY_ANDROID
_gameId = _androidGameId;
#elif UNITY_EDITOR
_gameId = _androidGameId; //Only for testing the functionality in the Editor
#endif
if (!Advertisement.isInitialized && Advertisement.isSupported)
{
Advertisement.Initialize(_gameId, _testMode, this);
}
}
public void OnInitializationComplete()
{
Debug.Log("Unity Ads initialization complete.");
}
public void OnInitializationFailed(UnityAdsInitializationError error, string message)
{
Debug.Log($"Unity Ads Initialization Failed: {error.ToString()} - {message}");
}
}
클래스 안의 내용을 복붙해주고
using UnityEngine.Advertisements;
IUnityAdsInitializationListener 인터페이스도 잊지 마세요.
아까 받은 키값을 입력해주면 됩니다.
Apple ID가 IOS ID이구요, Android ID가 Google ID 입니다. 주의하세요!
플레이해보면 콘솔창에 광고 초기화가 완료됐다고 뜨네요.
광고 띄우기
다음은 'Implementing rewarded ads in Unity'를 보겠습니다.
버튼을 클릭하면 광고가 보이게 해주고, 광고가 끝나면 보상을 주는 방식입니다.
코드▼
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Advertisements;
public class RewardedButton : MonoBehaviour, IUnityAdsLoadListener, IUnityAdsShowListener
{
[SerializeField] Button _showAdButton;
[SerializeField] string _androidAdUnitId = "Rewarded_Android";
[SerializeField] string _iOSAdUnitId = "Rewarded_iOS";
string _adUnitId = null; // This will remain null for unsupported platforms
void Awake()
{
// Get the Ad Unit ID for the current platform:
#if UNITY_IOS
_adUnitId = _iOSAdUnitId;
#elif UNITY_ANDROID
_adUnitId = _androidAdUnitId;
#endif
// Disable the button until the ad is ready to show:
_showAdButton.interactable = false;
}
// Call this public method when you want to get an ad ready to show.
public void LoadAd()
{
// IMPORTANT! Only load content AFTER initialization (in this example, initialization is handled in a different script).
Debug.Log("Loading Ad: " + _adUnitId);
Advertisement.Load(_adUnitId, this);
}
// If the ad successfully loads, add a listener to the button and enable it:
public void OnUnityAdsAdLoaded(string adUnitId)
{
Debug.Log("Ad Loaded: " + adUnitId);
if (adUnitId.Equals(_adUnitId))
{
// Configure the button to call the ShowAd() method when clicked:
_showAdButton.onClick.AddListener(ShowAd);
// Enable the button for users to click:
_showAdButton.interactable = true;
}
}
// Implement a method to execute when the user clicks the button:
public void ShowAd()
{
// Disable the button:
_showAdButton.interactable = false;
// Then show the ad:
Advertisement.Show(_adUnitId, this);
}
// Implement the Show Listener's OnUnityAdsShowComplete callback method to determine if the user gets a reward:
public void OnUnityAdsShowComplete(string adUnitId, UnityAdsShowCompletionState showCompletionState)
{
if (adUnitId.Equals(_adUnitId) && showCompletionState.Equals(UnityAdsShowCompletionState.COMPLETED))
{
Debug.Log("Unity Ads Rewarded Ad Completed");
// Grant a reward.
}
}
// Implement Load and Show Listener error callbacks:
public void OnUnityAdsFailedToLoad(string adUnitId, UnityAdsLoadError error, string message)
{
Debug.Log($"Error loading Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Use the error details to determine whether to try to load another ad.
}
public void OnUnityAdsShowFailure(string adUnitId, UnityAdsShowError error, string message)
{
Debug.Log($"Error showing Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Use the error details to determine whether to try to load another ad.
}
public void OnUnityAdsShowStart(string adUnitId) { }
public void OnUnityAdsShowClick(string adUnitId) { }
void OnDestroy()
{
// Clean up the button listeners:
_showAdButton.onClick.RemoveAllListeners();
}
}
클래스 안의 내용을 복붙해주고
using UnityEngine.UI;
using UnityEngine.Advertisements;
IUnityAdsLoadListener
IUnityAdsShowListener 인터페이스도 잊지 마세요.
// Implement the Show Listener's OnUnityAdsShowComplete callback method to determine if the user gets a reward:
public void OnUnityAdsShowComplete(string adUnitId, UnityAdsShowCompletionState showCompletionState)
{
if (adUnitId.Equals(_adUnitId) && showCompletionState.Equals(UnityAdsShowCompletionState.COMPLETED))
{
Debug.Log("Unity Ads Rewarded Ad Completed");
// Grant a reward.
}
}
이 부분이 광고를 다 보고 보상을 주는 함수입니다.
// Grant a reward.
SceneManager.LoadScene("MainScene");
이 광고를 넣을 게임의 방식은 게임이 끝나고 '끝'을 누르면 게임이 재시작되기 때문에
'끝'을 누르면 광고를 다 보고 다시 게임이 시작되도록 보상 밑에 해당 코드를 넣었습니다.
그리고 몇가지 수정을 좀 하겠습니다.
[SerializeField] Button _showAdButton;
이 부분은 지우고, 관련된 코드들도 다 지웠습니다.
지우고 난 후 코드▼
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.Advertisements;
using UnityEngine.SceneManagement;
public class RewardedButton : MonoBehaviour, IUnityAdsLoadListener, IUnityAdsShowListener
{
[SerializeField] string _androidAdUnitId = "Rewarded_Android";
[SerializeField] string _iOSAdUnitId = "Rewarded_iOS";
string _adUnitId = null; // This will remain null for unsupported platforms
void Awake()
{
// Get the Ad Unit ID for the current platform:
#if UNITY_IOS
_adUnitId = _iOSAdUnitId;
#elif UNITY_ANDROID
_adUnitId = _androidAdUnitId;
#endif
}
// Call this public method when you want to get an ad ready to show.
public void LoadAd()
{
// IMPORTANT! Only load content AFTER initialization (in this example, initialization is handled in a different script).
Debug.Log("Loading Ad: " + _adUnitId);
Advertisement.Load(_adUnitId, this);
}
// If the ad successfully loads, add a listener to the button and enable it:
public void OnUnityAdsAdLoaded(string adUnitId)
{
Debug.Log("Ad Loaded: " + adUnitId);
if (adUnitId.Equals(_adUnitId))
{
}
}
// Implement a method to execute when the user clicks the button:
public void ShowAd()
{
// Then show the ad:
Advertisement.Show(_adUnitId, this);
}
// Implement the Show Listener's OnUnityAdsShowComplete callback method to determine if the user gets a reward:
public void OnUnityAdsShowComplete(string adUnitId, UnityAdsShowCompletionState showCompletionState)
{
if (adUnitId.Equals(_adUnitId) && showCompletionState.Equals(UnityAdsShowCompletionState.COMPLETED))
{
Debug.Log("Unity Ads Rewarded Ad Completed");
// Grant a reward.
SceneManager.LoadScene("MainScene");
}
}
// Implement Load and Show Listener error callbacks:
public void OnUnityAdsFailedToLoad(string adUnitId, UnityAdsLoadError error, string message)
{
Debug.Log($"Error loading Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Use the error details to determine whether to try to load another ad.
}
public void OnUnityAdsShowFailure(string adUnitId, UnityAdsShowError error, string message)
{
Debug.Log($"Error showing Ad Unit {adUnitId}: {error.ToString()} - {message}");
// Use the error details to determine whether to try to load another ad.
}
public void OnUnityAdsShowStart(string adUnitId) { }
public void OnUnityAdsShowClick(string adUnitId) { }
void OnDestroy()
{
}
}
'끝'이라는 텍스트 오브젝트에 버튼을 추가했습니다.
실행해보면 '끝' 버튼을 눌렀을 때 광고 테스트창이 뜨는 것을 확인할 수 있습니다.
광고 테스트창이 뜨지 않고 오류가 발생한다면?▼
2024.04.11 - [Unity/오류] - [Unity] 광고 테스트창이 뜨지 않는 오류 - placementID
모바일로 빌드할 때 주의할 점은 'Test Mode'를 체크 해제하셔야 합니다.
체크되어 있으면 위에처럼 테스트창이 뜨고 광고가 나오지 않습니다.
댓글